之前写过Socket通信的TCP通信,所谓的TCP和UDP其实就是网络传输的一种传输协议,也就是网络传输的一种固有的传输方式。TCP协议,主要是用在一对多的情况下,需要有一个服务端来控制消息的传输。而UDP协议,则是用在多对多情况下,当然UDP也可以有服务端。但不管是那种方式,都有相应的应用场景。废话不多说,下面就上代码。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 |
using System.Collections.Generic; using System.Net; using System.Net.Sockets; using System.Text; using System.Threading; using UnityEngine; using System; public class UDPClient2Client : MonoBehaviour { public static UDPClient2Client Instance = null; private UdpClient client; private Thread thread = null; private IPEndPoint remotePoint; private string ip; private int port = 9090; public Action<string> receiveMsg = null; private string receiveString = null; void Awake() { if (Instance == null) { Instance = this; DontDestroyOnLoad(gameObject); } else { Destroy(gameObject); } } // Use this for initialization void Start() { ip = IPManager.ipAddress; remotePoint = new IPEndPoint(IPAddress.Any, 0); thread = new Thread(ReceiveMsg); thread.Start(); } void ReceiveMsg() { while (true) { client = new UdpClient(port); byte[] receiveData = client.Receive(ref remotePoint);//接收数据 receiveString = Encoding.UTF8.GetString(receiveData); client.Close(); } } void SendMsg(IPAddress ip, string _msg) { IPEndPoint remotePoint = new IPEndPoint(ip, port);//实例化一个远程端点 if (_msg != null) { byte[] sendData = Encoding.Default.GetBytes(_msg); UdpClient client = new UdpClient(); client.Send(sendData, sendData.Length, remotePoint);//将数据发送到远程端点 client.Close();//关闭连接 } } // Update is called once per frame void Update() { if (!string.IsNullOrEmpty(receiveString)) { //消息处理 if (receiveMsg != null && remotePoint.Address.ToString() != ip) { Debug.Log(remotePoint.Address + ":" + remotePoint.Port + " ---> " + receiveString); receiveMsg.Invoke(receiveString); receiveString = null; } } } void OnDestroy() { SocketQuit(); } void SocketQuit() { thread.Abort(); thread.Interrupt(); client.Close(); } void OnApplicationQuit() { SocketQuit(); } } |
当然,在这个代码里也要将传输过来的消息进行处理,和TCP的一样,要在主线程传输消息,否则会报错。UDP相对灵活一些,不需要服务器,只需要知道给谁发送消息,也就是ip。文章源自大腿Plus-https://www.zhaoshijun.com/archives/1157
之前写过在局域网内,不需要知道对方ip的情况发送消息(仅限PC端,移动也可以,但是没有具体的代码)文章源自大腿Plus-https://www.zhaoshijun.com/archives/1157
在Unity2018版本,不能直接获取局域网ip,下面是获取局域网ip的方法文章源自大腿Plus-https://www.zhaoshijun.com/archives/1157
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
using System.Net.NetworkInformation; using System.Net.Sockets; public class IPManager { public static string ipAddress { get { string output = ""; foreach (NetworkInterface item in NetworkInterface.GetAllNetworkInterfaces()) { #if UNITY_EDITOR_WIN || UNITY_STANDALONE_WIN NetworkInterfaceType _type1 = NetworkInterfaceType.Wireless80211; NetworkInterfaceType _type2 = NetworkInterfaceType.Ethernet; if ((item.NetworkInterfaceType == _type1 || item.NetworkInterfaceType == _type2) && item.OperationalStatus == OperationalStatus.Up) #endif { foreach (UnicastIPAddressInformation ip in item.GetIPProperties().UnicastAddresses) { //IPv4 if (ip.Address.AddressFamily == AddressFamily.InterNetwork) { output = ip.Address.ToString(); } } } } return output; } } } |
如果有什么问题可以加我QQ或者加入我的群一起讨论。文章源自大腿Plus-https://www.zhaoshijun.com/archives/1157 文章源自大腿Plus-https://www.zhaoshijun.com/archives/1157
我的微信
微信扫一扫
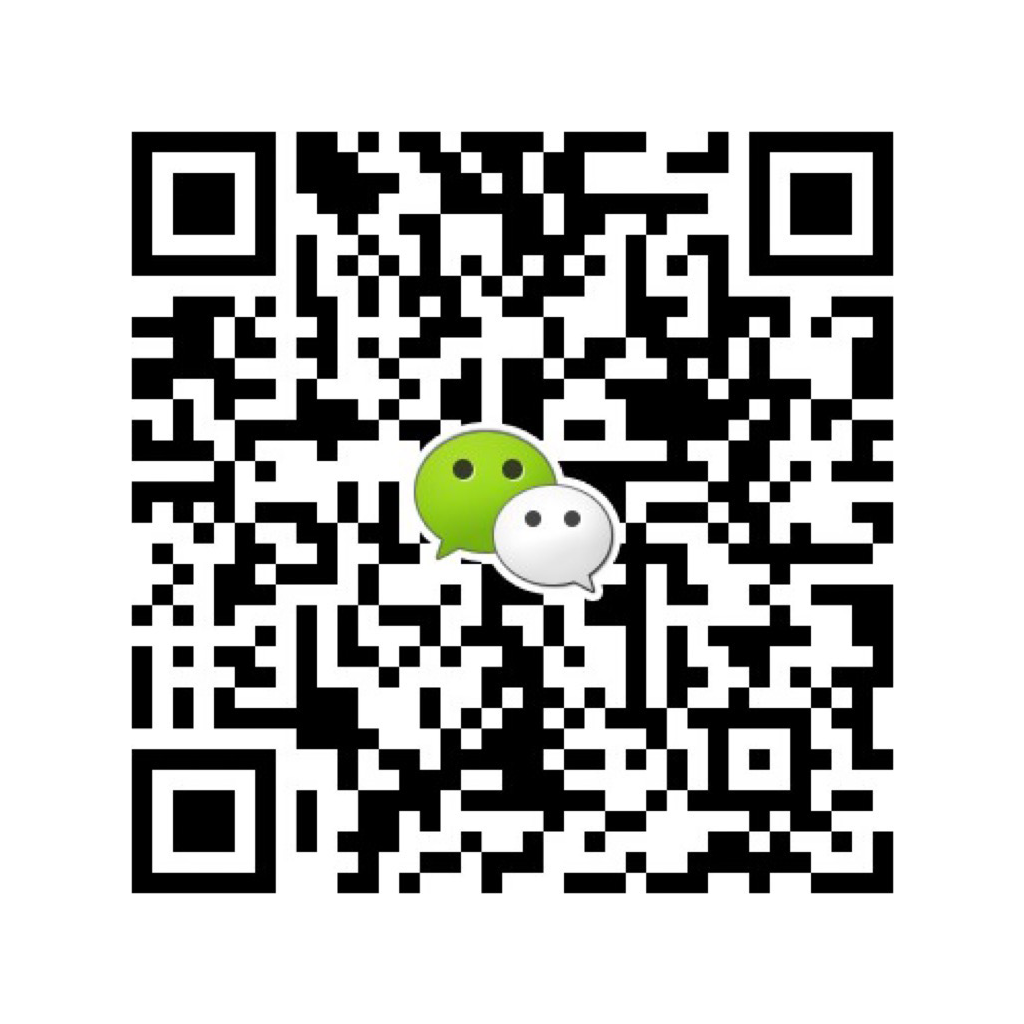
shijun_z
我的QQ
QQ扫一扫
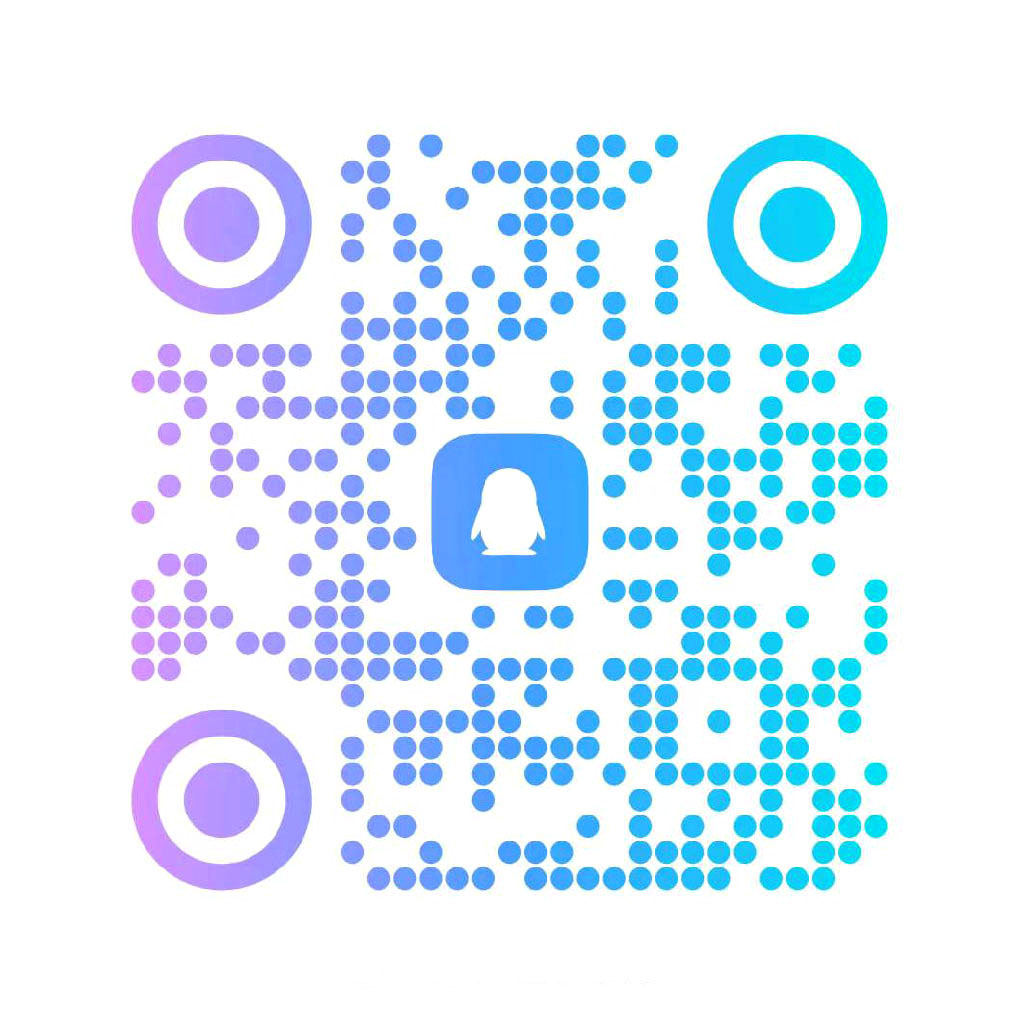
846207670
评论