在开发游戏的时候有时候会需要截屏分享,或者保存精彩时刻,需要用到截屏,我自己写了个截屏的插件,里面带有动画和声音,后面我将使用方法也放到下面。下面直接放主代码。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 |
using UnityEngine; using UnityEngine.UI; using System.Collections; using System.IO; using System; public class ScreenShot : MonoBehaviour { [HideInInspector] public string screenShotPath;//保存路径 [HideInInspector] public Size screenShotSize;//截屏的大小 [HideInInspector] private RawImage image;//截屏动画用 [HideInInspector] public AudioClip screenShotClip;//截屏声音 private Coroutine coroutine; [HideInInspector] public string imageName = ""; [HideInInspector] public Texture2D texture; // Use this for initialization void Start() { #if UNITY_ANDROID screenShotPath = "/mnt/sdcard/DCIM/Camera"; #endif #if UNITY_EDITOR screenShotPath = Application.dataPath + "/StreamingAssets/ScreenShotImage"; #endif //size = new Size(Screen.width, Screen.height);//默认截全屏 } // Update is called once per frame void Update() { } /// <summary> /// 截取屏幕像素,不带特效,默认大小 /// </summary> public void TakePhoto() { if (coroutine == null) { coroutine = StartCoroutine(Shot()); } } /// <summary> /// 截取屏幕像素,带特效,默认大小 /// </summary> /// <param name="effectParent"></param> public void TakePhoto(GameObject effectParent) { if (coroutine == null) { coroutine = StartCoroutine(Shot(effectParent)); } } /// <summary> /// 截取屏幕像素,不带特效,自定义大小 /// </summary> public void TakePhoto(Size size) { this.screenShotSize = size; if (coroutine == null) { coroutine = StartCoroutine(Shot()); } } /// <summary> /// 截取屏幕像素,带特效,自定义大小 /// </summary> /// <param name="effectParent"></param> public void TakePhoto(GameObject effectParent, Size size) { this.screenShotSize = size; if (coroutine == null) { coroutine = StartCoroutine(Shot(effectParent)); } } /// <summary> /// 截取某个相机渲染的画面,带特效,默认大小 /// </summary> /// <param name="cam"></param> /// <param name="effectParent"></param> public void TakePhoto(Camera cam, GameObject effectParent) { if (coroutine == null) { coroutine = StartCoroutine(Shot(cam, effectParent)); } } /// <summary> /// 截取某个相机渲染的画面,带特效,自定义大小 /// </summary> /// <param name="cam"></param> /// <param name="effectParent"></param> /// <param name="size"></param> public void TakePhoto(Camera cam, GameObject effectParent, Size size) { this.screenShotSize = size; if (coroutine == null) { coroutine = StartCoroutine(Shot(cam, effectParent)); } } /// <summary> /// 截取某个相机渲染的画面,不带特效,自定义大小 /// </summary> /// <param name="cam"></param> /// <param name="size"></param> public void TakePhoto(Camera cam, Size size) { this.screenShotSize = size; if (coroutine == null) { coroutine = StartCoroutine(Shot(cam)); } } /// <summary> /// 截取某个相机渲染的画面,不带特效,默认大小 /// </summary> /// <param name="cam"></param> public void TakePhoto(Camera cam) { if (coroutine == null) { coroutine = StartCoroutine(Shot(cam)); } } /// <summary> /// 截屏(截取某个相机渲染的画面,不带特效) /// </summary> /// <returns></returns> IEnumerator Shot(Camera camera) { texture = CaptureCamera(camera, new Rect(Screen.width / 2 - screenShotSize.w / 2, Screen.height / 2 - screenShotSize.h / 2, screenShotSize.w, screenShotSize.h)); yield return new WaitForEndOfFrame(); var img = texture.EncodeToPNG(); #if UNITY_EDITOR if (!Directory.Exists(screenShotPath))//创建生成目录,如果不存在则创建目录 { Directory.CreateDirectory(screenShotPath); } #endif imageName = string.Format("Image{0}.png", GetTimeStamp()); string file = Path.Combine(screenShotPath, imageName); File.WriteAllBytes(file, img); coroutine = null; } /// <summary> /// 截屏(截取某个相机渲染的画面,带特效) /// </summary> /// <returns></returns> IEnumerator Shot(Camera camera, GameObject effectParent) { texture = CaptureCamera(camera, new Rect(Screen.width / 2 - screenShotSize.w / 2, Screen.height / 2 - screenShotSize.h / 2, screenShotSize.w, screenShotSize.h)); yield return new WaitForEndOfFrame(); var img = texture.EncodeToPNG(); #if UNITY_EDITOR if (!Directory.Exists(screenShotPath))//创建生成目录,如果不存在则创建目录 { Directory.CreateDirectory(screenShotPath); } #endif imageName = string.Format("Image{0}.png", GetTimeStamp()); string file = Path.Combine(screenShotPath, imageName); File.WriteAllBytes(file, img); StartCoroutine(ShotEffect(texture, effectParent)); } /// <summary> /// 截屏(截取屏幕像素,不带特效) /// </summary> /// <returns></returns> IEnumerator Shot() { texture = new Texture2D(screenShotSize.w, screenShotSize.h, TextureFormat.RGB24, false); yield return new WaitForEndOfFrame(); texture.ReadPixels(new Rect(Screen.width / 2 - screenShotSize.w / 2, Screen.height / 2 - screenShotSize.h / 2, screenShotSize.w, screenShotSize.h), 0, 0, false); texture.Apply(); var img = texture.EncodeToPNG(); #if UNITY_EDITOR if (!Directory.Exists(screenShotPath))//创建生成目录,如果不存在则创建目录 { Directory.CreateDirectory(screenShotPath); } #endif imageName = string.Format("Image{0}.png", GetTimeStamp()); string file = Path.Combine(screenShotPath, imageName); File.WriteAllBytes(file, img); coroutine = null; } /// <summary> /// 截屏(截取屏幕像素,带特效) /// </summary> /// <returns></returns> IEnumerator Shot(GameObject effectParent) { texture = new Texture2D(screenShotSize.w, screenShotSize.h, TextureFormat.RGB24, false); yield return new WaitForEndOfFrame(); texture.ReadPixels(new Rect(Screen.width / 2 - screenShotSize.w / 2, Screen.height / 2 - screenShotSize.h / 2, screenShotSize.w, screenShotSize.h), 0, 0, false); texture.Apply(); var img = texture.EncodeToPNG(); #if UNITY_EDITOR if (!Directory.Exists(screenShotPath))//创建生成目录,如果不存在则创建目录 { Directory.CreateDirectory(screenShotPath); } #endif imageName = string.Format("Image{0}.png", GetTimeStamp()); string file = Path.Combine(screenShotPath, imageName); File.WriteAllBytes(file, img); StartCoroutine(ShotEffect(texture, effectParent)); } /// <summary> /// 截屏效果 /// </summary> /// <param name="texture"></param> /// <returns></returns> IEnumerator ShotEffect(Texture2D texture, GameObject effectParent) { float a = 1; float scale = 1; yield return new WaitForEndOfFrame(); image = new GameObject("ScreenShot").AddComponent<RawImage>(); AudioSource source = image.gameObject.AddComponent<AudioSource>(); if (screenShotClip != null) { source.clip = screenShotClip; source.loop = false; source.Play(); } image.transform.SetParent(FindObjectOfType<Canvas>().transform); image.transform.localPosition = Vector3.zero; image.rectTransform.sizeDelta = new Vector2(Screen.width, Screen.height); image.texture = texture; while (image.color.a > 0) { yield return new WaitForSeconds(0.02f); image.color = new Color(1, 1, 1, a -= 0.1f); image.transform.localScale = new Vector3(scale -= 0.01f, scale -= 0.01f, scale -= 0.01f); } Destroy(image.gameObject); coroutine = null; } /// <summary> /// 对相机截图。 /// </summary> /// <returns>The screenshot2.</returns> /// <param name="camera">Camera.要被截屏的相机</param> /// <param name="rect">Rect.截屏的区域</param> Texture2D CaptureCamera(Camera camera, Rect rect) { // 创建一个RenderTexture对象 RenderTexture rt = new RenderTexture(camera.pixelWidth, camera.pixelHeight, 0); // 临时设置相关相机的targetTexture为rt, 并手动渲染相关相机 camera.targetTexture = rt; camera.Render(); //ps: --- 如果这样加上第二个相机,可以实现只截图某几个指定的相机一起看到的图像。 //ps: camera2.targetTexture = rt; //ps: camera2.Render(); //ps: ------------------------------------------------------------------- // 激活这个rt, 并从中中读取像素。 RenderTexture.active = rt; Texture2D screenShot = new Texture2D((int)rect.width, (int)rect.height, TextureFormat.RGB24, false); screenShot.ReadPixels(rect, 0, 0);// 注:这个时候,它是从RenderTexture.active中读取像素 screenShot.Apply(); // 重置相关参数,以使用camera继续在屏幕上显示 camera.targetTexture = null; //ps: camera2.targetTexture = null; RenderTexture.active = null; // JC: added to avoid errors GameObject.Destroy(rt); return screenShot; } /// <summary> /// 获取时间戳,用来给保存的图片命名 /// </summary> /// <returns></returns> public static string GetTimeStamp() { TimeSpan ts = DateTime.UtcNow - new DateTime(1970, 1, 1, 0, 0, 0); return Convert.ToInt64(ts.TotalSeconds).ToString(); } } /// <summary> /// 大小 /// </summary> [Serializable] public struct Size { public int w; public int h; public Size(int width, int height) { w = width; h = height; } } |
只要将插件里预制体拖到场景里就可以,也可以将上面的脚本放到任意的GameObject上。文章源自大腿Plus-https://www.zhaoshijun.com/archives/437
文章源自大腿Plus-https://www.zhaoshijun.com/archives/437
这个代码我新增了对相机截图的功能,并写了好多重载的方法,用来做任意的截图操作,可对截屏的大小和有无特效效果传入不同的参数。个人对于相机截图比较实用,因为在截取是有些时候不需要UI的时候,可以将UI用一个单独的相机去渲染,其他想要截图的可以用另一个相机渲染,这样在截图的时候就不会把UI也截进去了。文章源自大腿Plus-https://www.zhaoshijun.com/archives/437
插件下载链接:http://pan.baidu.com/s/1jIxJBd8 密码:cw7w文章源自大腿Plus-https://www.zhaoshijun.com/archives/437 文章源自大腿Plus-https://www.zhaoshijun.com/archives/437
我的微信
微信扫一扫
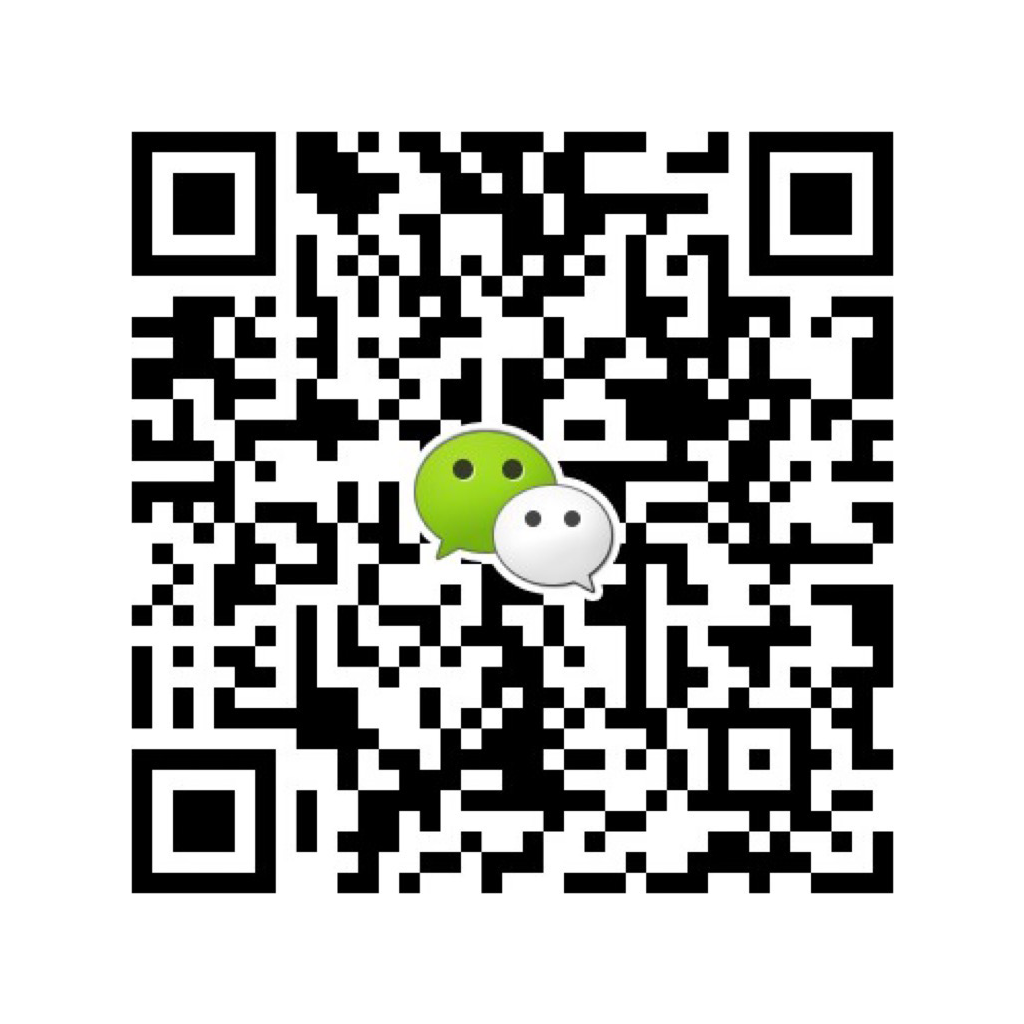
shijun_z
我的QQ
QQ扫一扫
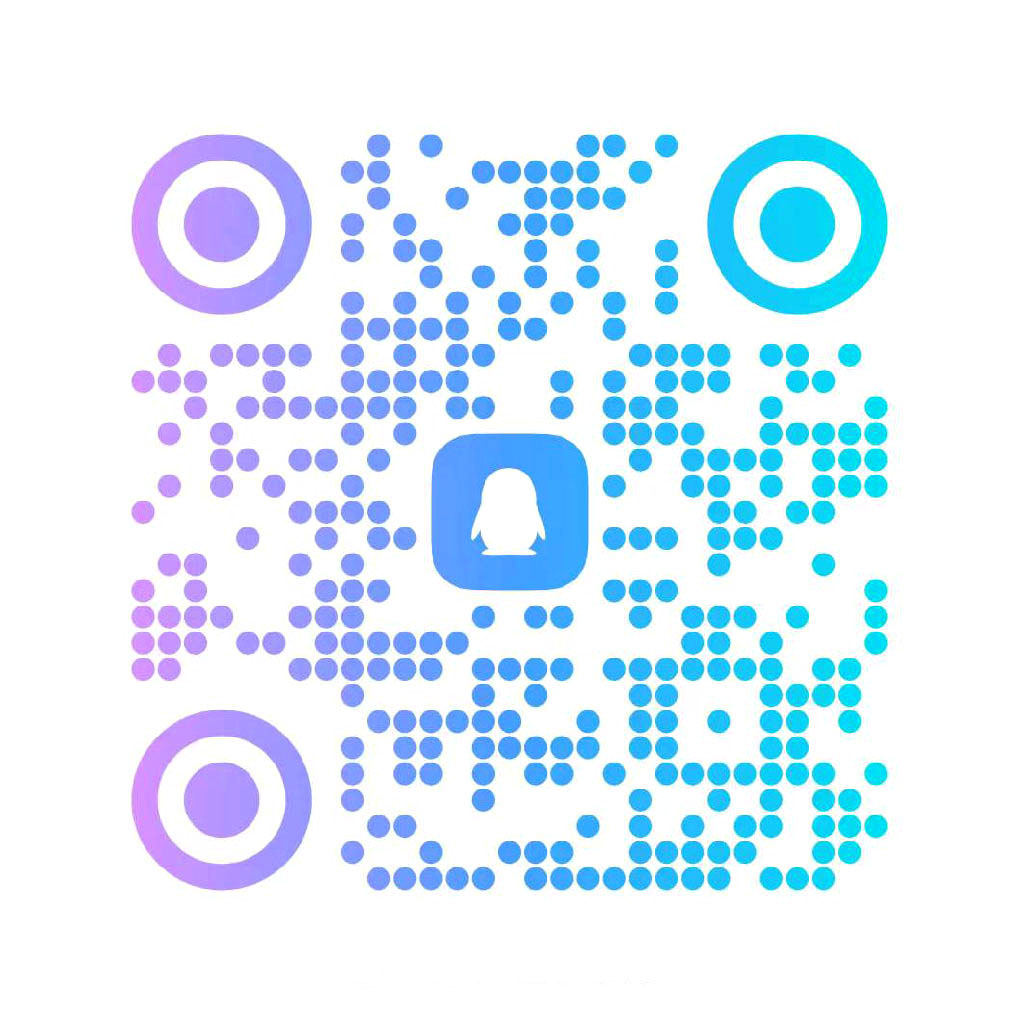
846207670
1F
很赞,不错!