在做聊天输入框的时候,UGUI自带的InputField只能设置输入内容显示最新输入的内容和输入固定长度的内容,并没有自适应内容改变输入框的长度和宽度,网上搜了一下,在蛮牛上看到一个能自适应长度的,可以根据内容改变输入框的长度,但是如果长度太长就会超出范围,后来我把代码改了一下,可以固定长度,然后自动换行,自适应宽度。
整体的思路就是继承UGUI提供的Layout接口,根据接口提供的方法计算出输入内容的宽高,接口方法就能够自适应宽高。下面是效果。文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
上面就是运行后的效果,下面就上代码。文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 |
using System.Collections; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using UnityEngine.EventSystems; [RequireComponent(typeof(InputField))] public class InputFieldScaler : MonoBehaviour, ILayoutElement { private Text textComponent { get { return this.GetComponent().textComponent; } } public TextGenerationSettings GetTextGenerationSettings(Vector2 extents) { var settings = textComponent.GetGenerationSettings(extents); settings.generateOutOfBounds = true; return settings; } private RectTransform m_Rect; private RectTransform rectTransform { get { if (m_Rect == null) m_Rect = GetComponent(); return m_Rect; } } public void OnValueChanged(string v) { if (!fixedWidth) { rectTransform.SetSizeWithCurrentAnchors((RectTransform.Axis)0, LayoutUtility.GetPreferredWidth(m_Rect)); } rectTransform.SetSizeWithCurrentAnchors((RectTransform.Axis)1, LayoutUtility.GetPreferredHeight(m_Rect)); } void OnEnable() { this.inputField.onValueChanged.AddListener(OnValueChanged); } void OnDisable() { } private Vector2 originalSize; private InputField _inputField; public InputField inputField { get { return _inputField ?? (_inputField = this.GetComponent()); } } private float _offsetHeight; public float offsetHeight { get { if (_offsetHeight == 0) _offsetHeight = generatorForLayout.GetPreferredHeight(text, GetTextGenerationSettings(Vector2.zero)) / textComponent.pixelsPerUnit; return _offsetHeight; } } private float _offsetTextComponentLeftRingt; public float offsetTextComponentLeftRingt { get { if (_offsetTextComponentLeftRingt == 0) _offsetTextComponentLeftRingt = Mathf.Abs(rectTransform.rect.width - textComponent.rectTransform.rect.width); return _offsetTextComponentLeftRingt; } } protected void Awake() { textComponent.fontSize = fontSize; inputField.placeholder.GetComponent().fontSize = fontSize; this.originalSize = this.GetComponent().sizeDelta; inputField.lineType = fixedWidth ? InputField.LineType.MultiLineNewline : InputField.LineType.SingleLine; rectTransform.SetSizeWithCurrentAnchors((RectTransform.Axis)1, LayoutUtility.GetPreferredHeight(m_Rect)); } private string text { get { return this.GetComponent().text; } } private TextGenerator _generatorForLayout; public TextGenerator generatorForLayout { get { return _generatorForLayout ?? (_generatorForLayout = new TextGenerator()); } } public void Update() { } public float preferredWidth { get { if (fixedWidth) { return this.originalSize.x; } else { if (keepInitWidthSize) { return Mathf.Max(this.originalSize.x, generatorForLayout.GetPreferredWidth(text, GetTextGenerationSettings(Vector2.zero)) / textComponent.pixelsPerUnit + offsetTextComponentLeftRingt); } else { return generatorForLayout.GetPreferredWidth(text, GetTextGenerationSettings(Vector2.zero)) / textComponent.pixelsPerUnit + offsetTextComponentLeftRingt; } } } } public virtual float preferredHeight { get { if (fixedWidth) { return generatorForLayout.GetPreferredHeight(text, GetTextGenerationSettings(new Vector2(this.textComponent.GetPixelAdjustedRect().size.x, 0.0f))) / textComponent.pixelsPerUnit + offsetHeight; } else { return generatorForLayout.GetPreferredHeight(text, GetTextGenerationSettings(new Vector2(this.textComponent.GetPixelAdjustedRect().size.x, 0.0f))) / textComponent.pixelsPerUnit + offsetHeight; } } } public virtual void CalculateLayoutInputHorizontal() { } public virtual void CalculateLayoutInputVertical() { } public virtual float minWidth { get { return -1; } } public virtual float minHeight { get { return -1; } } public virtual float flexibleWidth { get { return -1; } } public virtual float flexibleHeight { get { return -1; } } //[Tooltip("输入框的字体大小,InputField的大小会随字体大小改变高度")] [HideInInspector] public int fontSize = 20; //[Tooltip("是否保持InputField的宽度不变")] [HideInInspector] public bool fixedWidth = true; //[Tooltip("是否不限制InputField的宽度")] [HideInInspector] public bool keepInitWidthSize = true; //[SerializeField] //[Tooltip("提高Layout计算优先级,要比InputField大 这里设为1")] private int priority = 1; public virtual int layoutPriority { get { return priority; } } } |
代码其实很简单,就是通过输入的字符串的内容,在接口方法里计算出宽高就可以了,接口方法会被自动调用。文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
参考代码:http://www.manew.com/thread-107880-1-1.html文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
下载链接: https://pan.baidu.com/s/1ZpOQueUyUg1ef7LpJTKShw 提取码: tk8h文章源自大腿Plus-https://www.zhaoshijun.com/archives/814 文章源自大腿Plus-https://www.zhaoshijun.com/archives/814
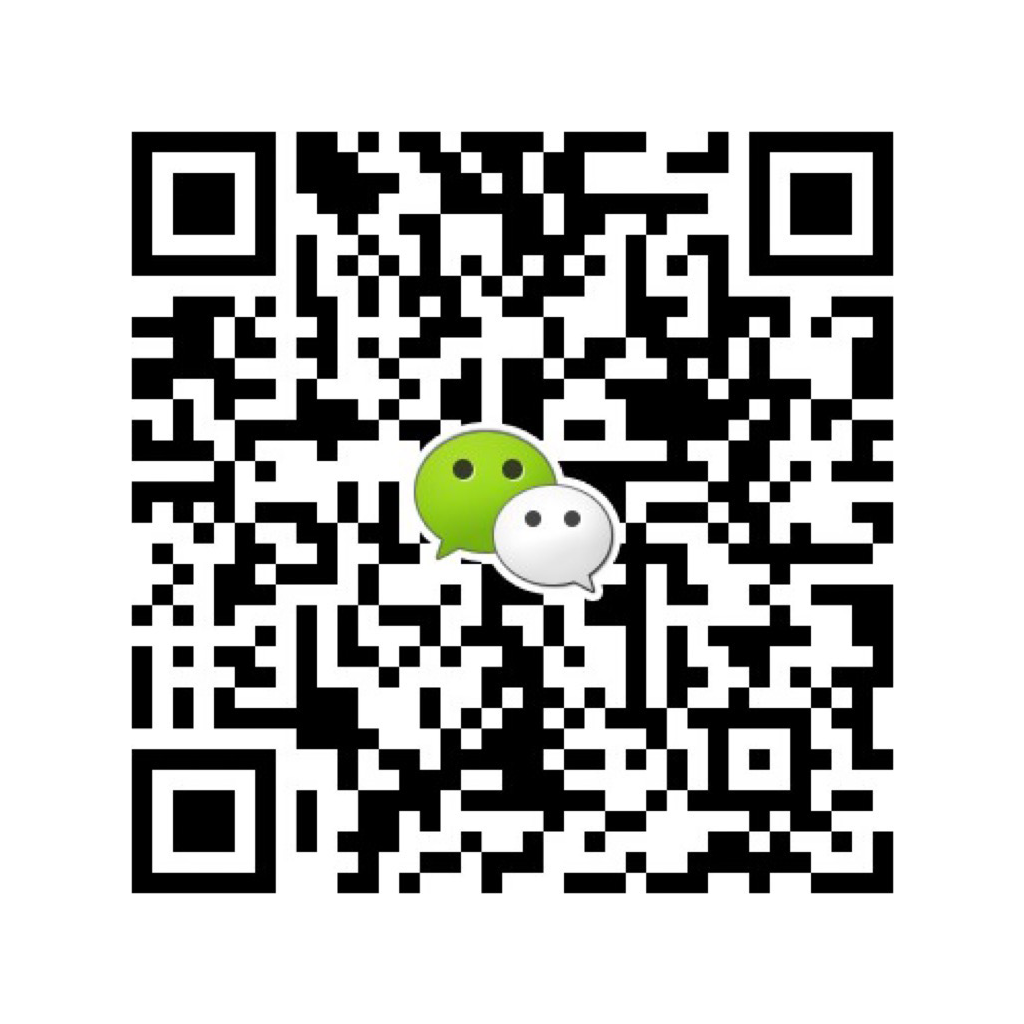
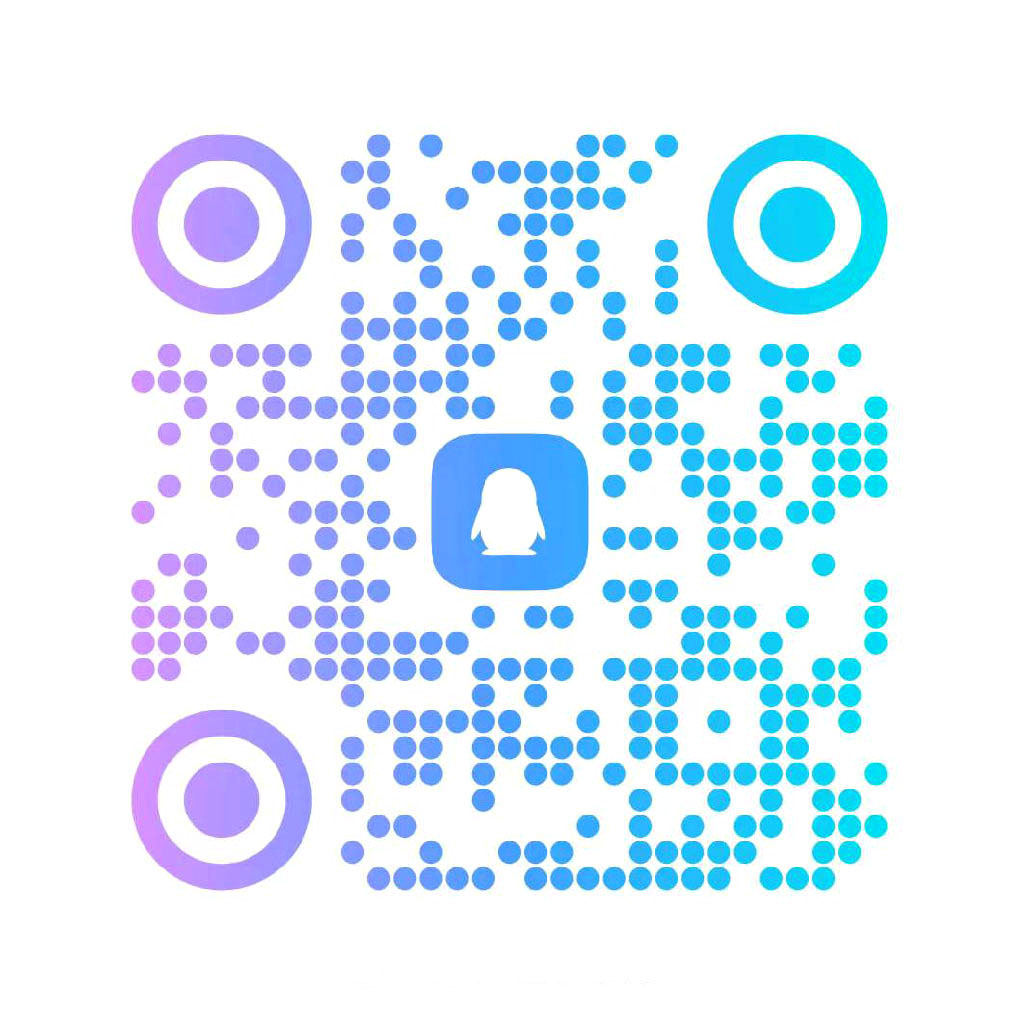
评论